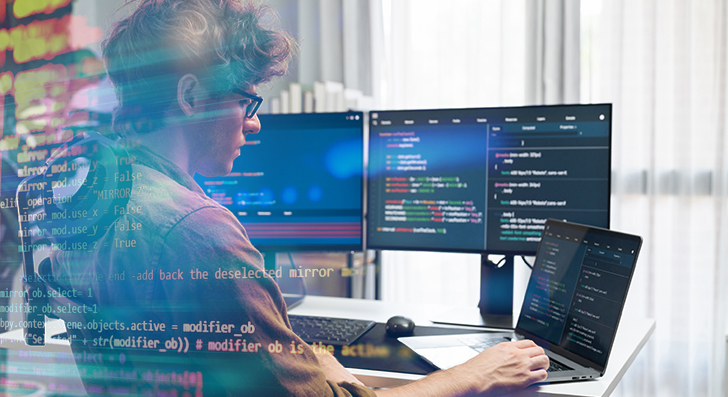
Scalability implies your software can tackle expansion—a lot more customers, more facts, plus much more targeted traffic—with no breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on afterwards—it should be section of the strategy from the start. Several purposes fall short if they grow speedy due to the fact the original structure can’t manage the additional load. Like a developer, you might want to Feel early regarding how your program will behave stressed.
Start off by designing your architecture to get flexible. Keep away from monolithic codebases where almost everything is tightly connected. Alternatively, use modular design or microservices. These designs split your application into more compact, unbiased parts. Just about every module or service can scale on its own devoid of affecting The entire process.
Also, think about your database from day just one. Will it require to manage one million customers or maybe 100? Choose the appropriate style—relational or NoSQL—according to how your knowledge will improve. Plan for sharding, indexing, and backups early, even if you don’t need to have them nonetheless.
An additional significant issue is to avoid hardcoding assumptions. Don’t create code that only operates beneath latest disorders. Give thought to what would happen In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that support scaling, like concept queues or function-driven programs. These enable your application cope with additional requests devoid of having overloaded.
After you Construct with scalability in mind, you're not just making ready for fulfillment—you might be cutting down long run complications. A effectively-planned system is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Database
Deciding on the appropriate database can be a essential Portion of developing scalable purposes. Not all databases are designed precisely the same, and using the wrong you can sluggish you down or perhaps result in failures as your app grows.
Get started by knowledge your info. Is it really structured, like rows in a very table? If Certainly, a relational databases like PostgreSQL or MySQL is a great suit. They are potent with associations, transactions, and consistency. Additionally they assistance scaling procedures like read through replicas, indexing, and partitioning to handle extra visitors and facts.
Should your details is much more adaptable—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing big volumes of unstructured or semi-structured facts and may scale horizontally additional effortlessly.
Also, take into consideration your study and generate designs. Are you accomplishing a lot of reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty produce load? Look into databases that will tackle large write throughput, as well as party-primarily based facts storage units like Apache Kafka (for short-term knowledge streams).
It’s also good to think ahead. You may not need to have Superior scaling capabilities now, but deciding on a database that supports them implies you received’t have to have to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your information according to your entry styles. And generally monitor database overall performance while you improve.
To put it briefly, the right database will depend on your application’s structure, pace demands, And just how you be expecting it to improve. Choose time to select sensibly—it’ll help you save loads of problems later on.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, every single modest hold off provides up. Badly prepared code or unoptimized queries can slow down effectiveness and overload your process. That’s why it’s essential to Make successful logic from the start.
Begin by creating thoroughly clean, simple code. Avoid repeating logic and take away nearly anything avoidable. Don’t pick the most sophisticated Answer if a straightforward just one performs. Keep your capabilities limited, targeted, and straightforward to check. Use profiling resources to seek out bottlenecks—locations where by your code normally takes as well extensive to operate or makes use of too much memory.
Upcoming, examine your databases queries. These usually slow things down in excess of the code itself. Ensure that Every question only asks for the data you really need. Prevent Choose *, which fetches anything, and rather pick out precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, especially across substantial tables.
In the event you see the identical details currently being asked for time and again, use caching. Store the outcome quickly utilizing instruments like Redis or Memcached so you don’t really need to repeat highly-priced operations.
Also, batch your database functions any time you can. As opposed to updating a row one after the other, update them in groups. This cuts down on overhead and can make your application more effective.
Remember to take a look at with large datasets. Code and queries that perform great with 100 data could possibly crash whenever they have to manage 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when required. These actions support your software keep sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your app grows, it's to deal with far more people plus more traffic. If every thing goes via a single server, it's going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two instruments enable keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across numerous servers. Rather than 1 server performing all of the work, the load balancer routes users to distinct servers depending on availability. This means no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to setup.
Caching is about storing information quickly so it might be reused speedily. When customers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You'll be able to read more provide it through the cache.
There are 2 common different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static data files close to the person.
Caching minimizes databases load, enhances speed, and can make your app far more effective.
Use caching for things which don’t change generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are simple but strong equipment. Alongside one another, they help your application tackle much more people, continue to be quick, and Get well from complications. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable programs, you need resources that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you need them. You don’t need to acquire hardware or guess potential capability. When website traffic boosts, you could increase more resources with just a few clicks or automatically using auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also offer you expert services like managed databases, storage, load balancing, and stability instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your application and every little thing it has to run—code, libraries, configurations—into just one device. This can make it effortless to move your app concerning environments, from the laptop towards the cloud, without surprises. Docker is the preferred Device for this.
When your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and Restoration. If 1 element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You can update or scale pieces independently, that's great for effectiveness and reliability.
To put it briefly, making use of cloud and container applications signifies you can scale quick, deploy quickly, and Recuperate promptly when difficulties materialize. If you'd like your application to develop devoid of limits, start out using these equipment early. They help you save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Monitor Anything
In the event you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of setting up scalable systems.
Commence by monitoring primary metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and solutions are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Regulate how much time it's going to take for users to load pages, how often errors occur, and exactly where they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential difficulties. As an example, Should your response time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This assists you fix issues speedy, normally in advance of people even observe.
Monitoring can also be useful after you make modifications. If you deploy a completely new element and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, visitors and details raise. Without having monitoring, you’ll miss out on signs of hassle until eventually it’s also late. But with the right applications in position, you continue to be in control.
In short, checking will help you keep your application responsible and scalable. It’s not almost spotting failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for major organizations. Even compact apps will need a strong Basis. By designing meticulously, optimizing wisely, and using the suitable tools, you may build apps that improve smoothly devoid of breaking stressed. Commence smaller, think massive, and Establish intelligent.